Chapter 41 Messmer human ESC (Smart-seq2)
41.1 Introduction
This performs an analysis of the human embryonic stem cell (hESC) dataset generated with Smart-seq2 (Messmer et al. 2019), which contains several plates of naive and primed hESCs. The chapter’s code is based on the steps in the paper’s GitHub repository, with some additional steps for cell cycle effect removal contributed by Philippe Boileau.
41.2 Data loading
Converting the batch to a factor, to make life easier later on.
41.3 Quality control
Let’s have a look at the QC statistics.
## low_lib_size low_n_features high_subsets_Mito_percent
## 107 99 22
## high_altexps_ERCC_percent discard
## 117 156
gridExtra::grid.arrange(
plotColData(original, x="experiment batch", y="sum",
colour_by=I(filtered$discard), other_field="phenotype") +
facet_wrap(~phenotype) + scale_y_log10(),
plotColData(original, x="experiment batch", y="detected",
colour_by=I(filtered$discard), other_field="phenotype") +
facet_wrap(~phenotype) + scale_y_log10(),
plotColData(original, x="experiment batch", y="subsets_Mito_percent",
colour_by=I(filtered$discard), other_field="phenotype") +
facet_wrap(~phenotype),
plotColData(original, x="experiment batch", y="altexps_ERCC_percent",
colour_by=I(filtered$discard), other_field="phenotype") +
facet_wrap(~phenotype),
ncol=1
)
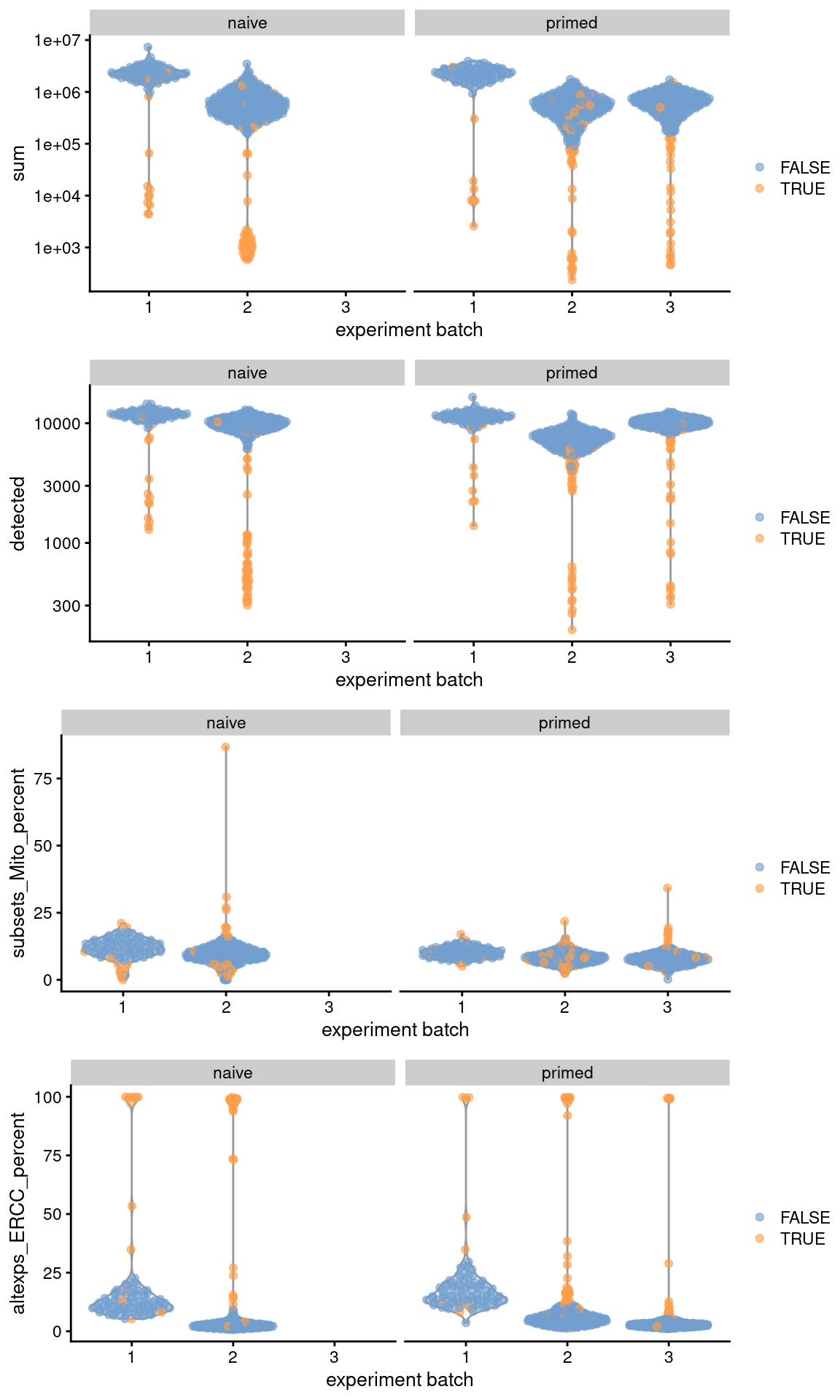
Figure 41.1: Distribution of QC metrics across batches (x-axis) and phenotypes (facets) for cells in the Messmer hESC dataset. Each point is a cell and is colored by whether it was discarded.
41.4 Normalization
library(scran)
set.seed(10000)
clusters <- quickCluster(sce.mess)
sce.mess <- computeSumFactors(sce.mess, cluster=clusters)
sce.mess <- logNormCounts(sce.mess)
par(mfrow=c(1,2))
plot(sce.mess$sum, sizeFactors(sce.mess), log = "xy", pch=16,
xlab = "Library size (millions)", ylab = "Size factor",
col = ifelse(sce.mess$phenotype == "naive", "black", "grey"))
spike.sf <- librarySizeFactors(altExp(sce.mess, "ERCC"))
plot(sizeFactors(sce.mess), spike.sf, log = "xy", pch=16,
ylab = "Spike-in size factor", xlab = "Deconvolution size factor",
col = ifelse(sce.mess$phenotype == "naive", "black", "grey"))
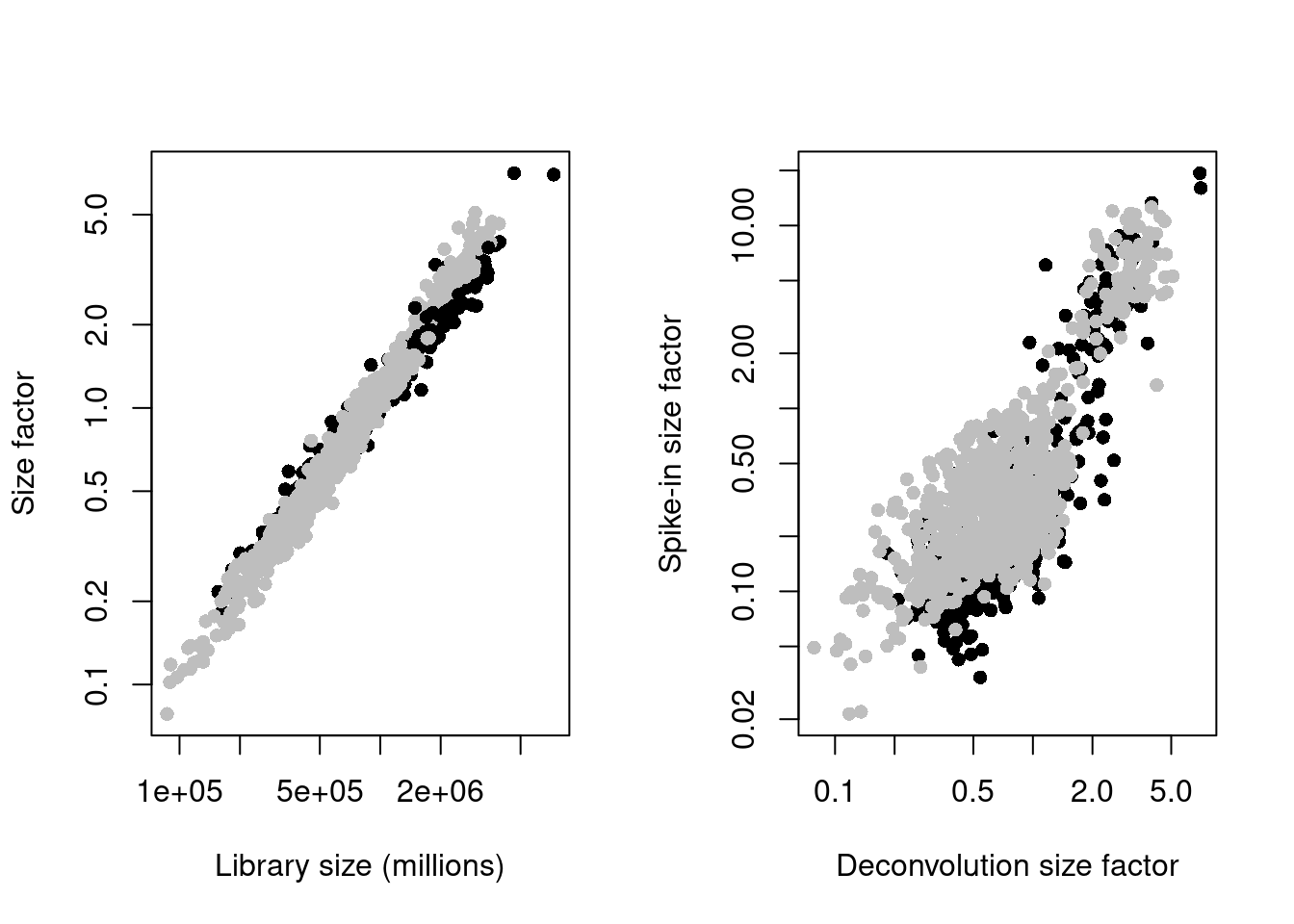
Figure 41.2: Deconvolution size factors plotted against the library size (left) and spike-in size factors plotted against the deconvolution size factors (right). Each point is a cell and is colored by its phenotype.
41.5 Cell cycle phase assignment
Here, we use multiple cores to speed up the processing.
set.seed(10001)
hs_pairs <- readRDS(system.file("exdata", "human_cycle_markers.rds", package="scran"))
assigned <- cyclone(sce.mess, pairs=hs_pairs,
gene.names=rownames(sce.mess),
BPPARAM=BiocParallel::MulticoreParam(10))
sce.mess$phase <- assigned$phases
##
## G1 G2M S
## 460 406 322
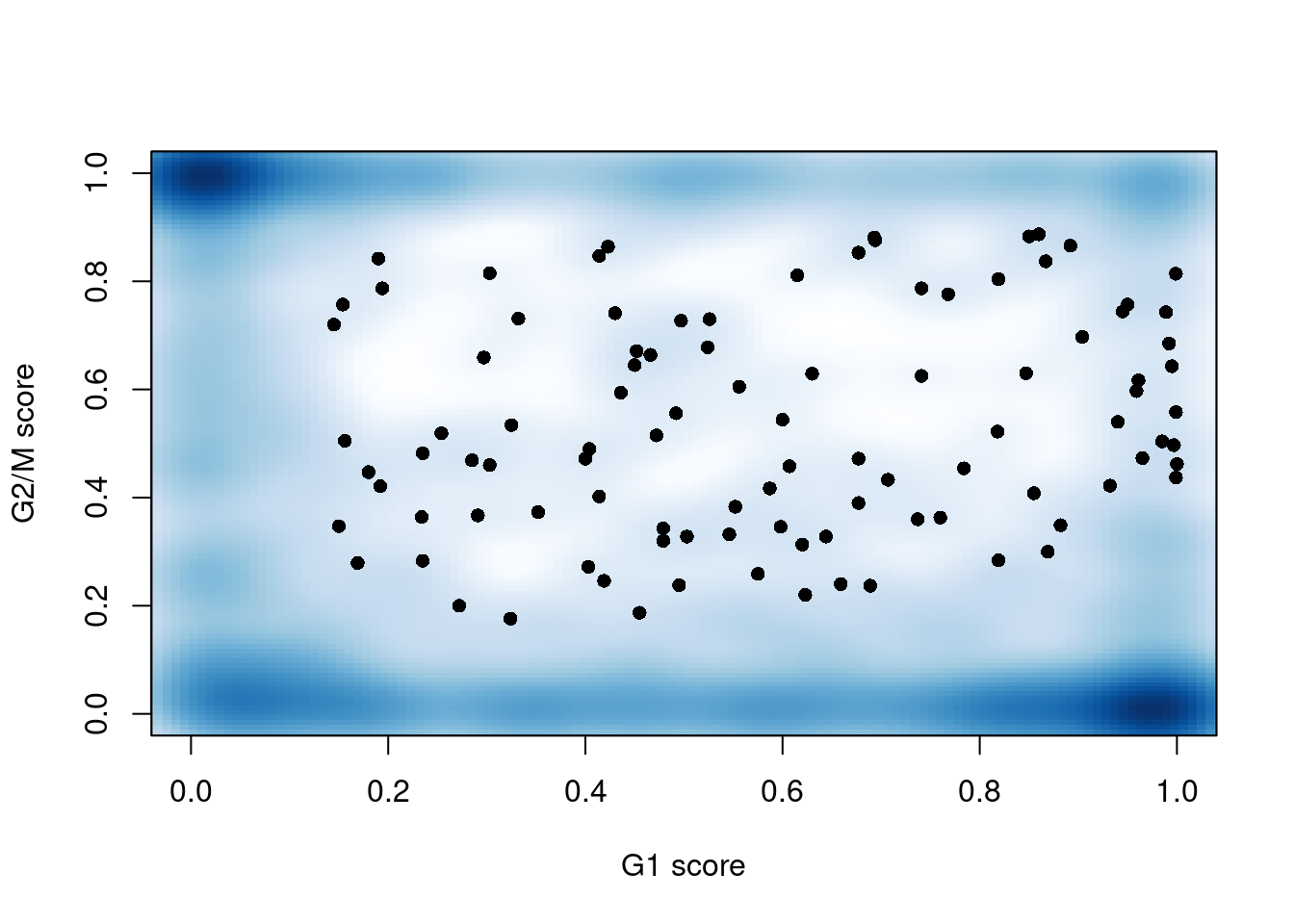
Figure 41.3: G1 cyclone()
phase scores against the G2/M phase scores for each cell in the Messmer hESC dataset.
41.6 Feature selection
dec <- modelGeneVarWithSpikes(sce.mess, "ERCC", block = sce.mess$`experiment batch`)
top.hvgs <- getTopHVGs(dec, prop = 0.1)
par(mfrow=c(1,3))
for (i in seq_along(dec$per.block)) {
current <- dec$per.block[[i]]
plot(current$mean, current$total, xlab="Mean log-expression",
ylab="Variance", pch=16, cex=0.5, main=paste("Batch", i))
fit <- metadata(current)
points(fit$mean, fit$var, col="red", pch=16)
curve(fit$trend(x), col='dodgerblue', add=TRUE, lwd=2)
}

Figure 41.4: Per-gene variance of the log-normalized expression values in the Messmer hESC dataset, plotted against the mean for each batch. Each point represents a gene with spike-ins shown in red and the fitted trend shown in blue.
41.7 Batch correction
We eliminate the obvious batch effect between batches with linear regression, which is possible due to the replicated nature of the experimental design.
We set keep=1:2
to retain the effect of the first two coefficients in design
corresponding to our phenotype of interest.
41.8 Dimensionality Reduction
We could have set d=
and subset.row=
in correctExperiments()
to automatically perform a PCA on the the residual matrix with the subset of HVGs,
but we’ll just explicitly call runPCA()
here to keep things simple.
set.seed(1101001)
sce.mess <- runPCA(sce.mess, subset_row = top.hvgs, exprs_values = "corrected")
sce.mess <- runTSNE(sce.mess, dimred = "PCA", perplexity = 40)
From a naive PCA, the cell cycle appears to be a major source of biological variation within each phenotype.
gridExtra::grid.arrange(
plotTSNE(sce.mess, colour_by = "phenotype") + ggtitle("By phenotype"),
plotTSNE(sce.mess, colour_by = "experiment batch") + ggtitle("By batch "),
plotTSNE(sce.mess, colour_by = "CDK1", swap_rownames="SYMBOL") + ggtitle("By CDK1"),
plotTSNE(sce.mess, colour_by = "phase") + ggtitle("By phase"),
ncol = 2
)
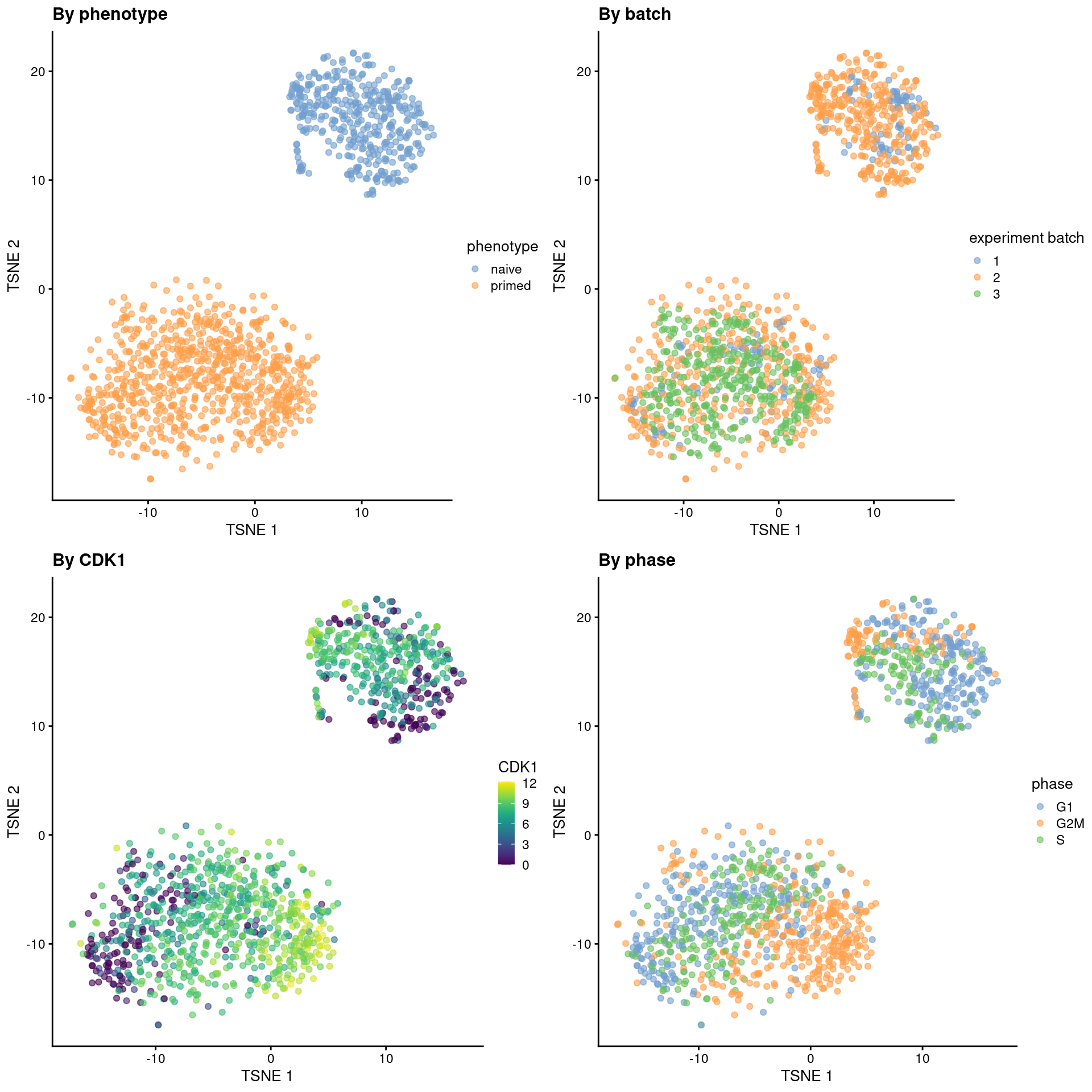
Figure 41.5: Obligatory \(t\)-SNE plots of the Messmer hESC dataset, where each point is a cell and is colored by various attributes.
We perform contrastive PCA (cPCA) and sparse cPCA (scPCA) on the corrected log-expression data to obtain the same number of PCs. Given that the naive hESCs are actually reprogrammed primed hESCs, we will use the single batch of primed-only hESCs as the “background” dataset to remove the cell cycle effect.
library(scPCA)
is.bg <- sce.mess$`experiment batch`=="3"
target <- sce.mess[,!is.bg]
background <- sce.mess[,is.bg]
mat.target <- t(assay(target, "corrected")[top.hvgs,])
mat.background <- t(assay(background, "corrected")[top.hvgs,])
set.seed(1010101001)
con_out <- scPCA(
target = mat.target,
background = mat.background,
penalties = 0, # no penalties = non-sparse cPCA.
n_eigen = 50,
contrasts = 100
)
reducedDim(target, "cPCA") <- con_out$x
set.seed(101010101)
sparse_con_out <- scPCA(
target = mat.target,
background = mat.background,
penalties = 1e-4,
n_eigen = 50,
contrasts = 100,
alg = "rand_var_proj" # for speed.
)
reducedDim(target, "scPCA") <- sparse_con_out$x
We see greater intermingling between phases within both the naive and primed cells after cPCA and scPCA.
set.seed(1101001)
target <- runTSNE(target, dimred = "cPCA", perplexity = 40, name="cPCA+TSNE")
target <- runTSNE(target, dimred = "scPCA", perplexity = 40, name="scPCA+TSNE")
gridExtra::grid.arrange(
plotReducedDim(target, "cPCA+TSNE", colour_by = "phase") + ggtitle("After cPCA"),
plotReducedDim(target, "scPCA+TSNE", colour_by = "phase") + ggtitle("After scPCA"),
ncol=2
)
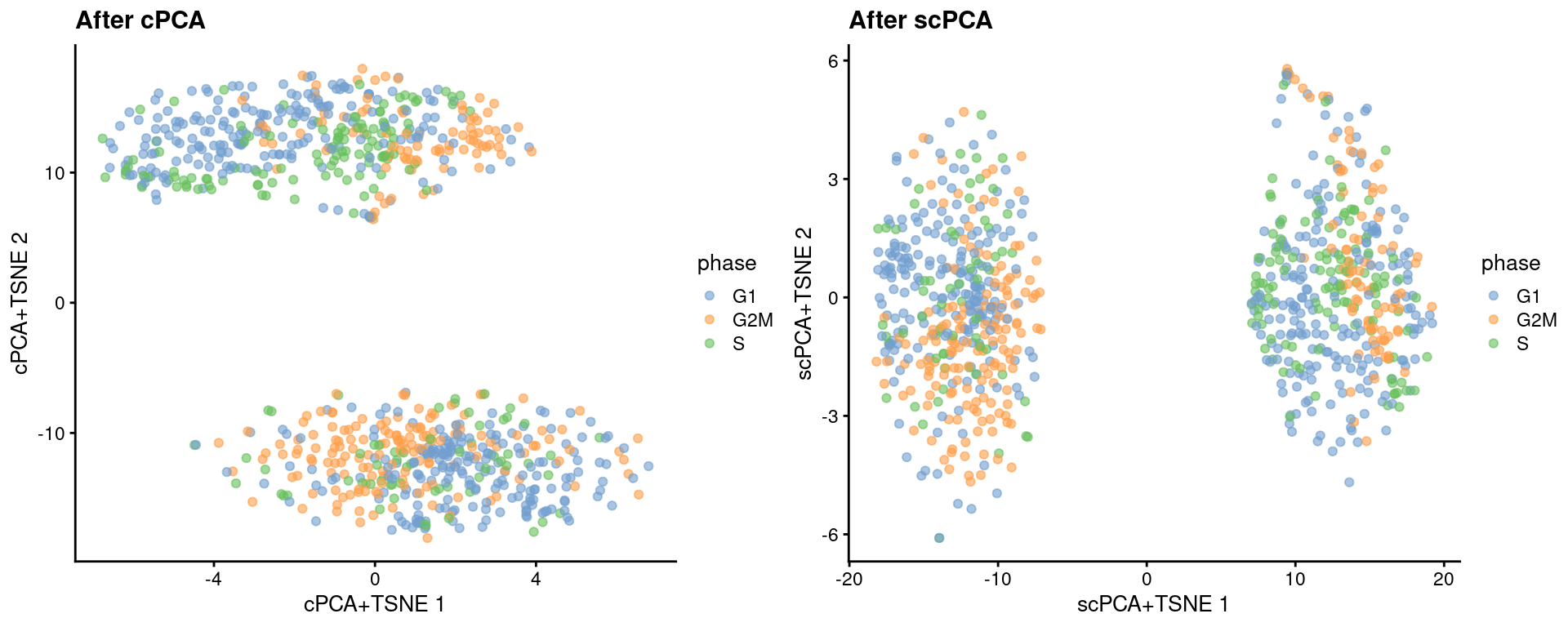
Figure 41.6: More \(t\)-SNE plots of the Messmer hESC dataset after cPCA and scPCA, where each point is a cell and is colored by its assigned cell cycle phase.
We can quantify the change in the separation between phases within each phenotype using the silhouette coefficient.
library(bluster)
naive <- target[,target$phenotype=="naive"]
primed <- target[,target$phenotype=="primed"]
N <- approxSilhouette(reducedDim(naive, "PCA"), naive$phase)
P <- approxSilhouette(reducedDim(primed, "PCA"), primed$phase)
c(naive=mean(N$width), primed=mean(P$width))
## naive primed
## 0.02032 0.03025
cN <- approxSilhouette(reducedDim(naive, "cPCA"), naive$phase)
cP <- approxSilhouette(reducedDim(primed, "cPCA"), primed$phase)
c(naive=mean(cN$width), primed=mean(cP$width))
## naive primed
## 0.007696 0.011941
scN <- approxSilhouette(reducedDim(naive, "scPCA"), naive$phase)
scP <- approxSilhouette(reducedDim(primed, "scPCA"), primed$phase)
c(naive=mean(scN$width), primed=mean(scP$width))
## naive primed
## 0.006614 0.014601
Session Info
R version 4.0.4 (2021-02-15)
Platform: x86_64-pc-linux-gnu (64-bit)
Running under: Ubuntu 20.04.2 LTS
Matrix products: default
BLAS: /home/biocbuild/bbs-3.12-books/R/lib/libRblas.so
LAPACK: /home/biocbuild/bbs-3.12-books/R/lib/libRlapack.so
locale:
[1] LC_CTYPE=en_US.UTF-8 LC_NUMERIC=C
[3] LC_TIME=en_US.UTF-8 LC_COLLATE=C
[5] LC_MONETARY=en_US.UTF-8 LC_MESSAGES=en_US.UTF-8
[7] LC_PAPER=en_US.UTF-8 LC_NAME=C
[9] LC_ADDRESS=C LC_TELEPHONE=C
[11] LC_MEASUREMENT=en_US.UTF-8 LC_IDENTIFICATION=C
attached base packages:
[1] parallel stats4 stats graphics grDevices utils datasets
[8] methods base
other attached packages:
[1] bluster_1.0.0 scPCA_1.4.0
[3] batchelor_1.6.2 scran_1.18.5
[5] scater_1.18.6 ggplot2_3.3.3
[7] AnnotationHub_2.22.0 BiocFileCache_1.14.0
[9] dbplyr_2.1.0 ensembldb_2.14.0
[11] AnnotationFilter_1.14.0 GenomicFeatures_1.42.2
[13] AnnotationDbi_1.52.0 scRNAseq_2.4.0
[15] SingleCellExperiment_1.12.0 SummarizedExperiment_1.20.0
[17] Biobase_2.50.0 GenomicRanges_1.42.0
[19] GenomeInfoDb_1.26.4 IRanges_2.24.1
[21] S4Vectors_0.28.1 BiocGenerics_0.36.0
[23] MatrixGenerics_1.2.1 matrixStats_0.58.0
[25] BiocStyle_2.18.1 rebook_1.0.0
loaded via a namespace (and not attached):
[1] igraph_1.2.6 lazyeval_0.2.2
[3] listenv_0.8.0 BiocParallel_1.24.1
[5] digest_0.6.27 htmltools_0.5.1.1
[7] viridis_0.5.1 fansi_0.4.2
[9] magrittr_2.0.1 memoise_2.0.0
[11] cluster_2.1.0 limma_3.46.0
[13] globals_0.14.0 Biostrings_2.58.0
[15] askpass_1.1 prettyunits_1.1.1
[17] colorspace_2.0-0 blob_1.2.1
[19] rappdirs_0.3.3 rbibutils_2.0
[21] xfun_0.22 dplyr_1.0.5
[23] callr_3.5.1 crayon_1.4.1
[25] RCurl_1.98-1.3 jsonlite_1.7.2
[27] graph_1.68.0 glue_1.4.2
[29] gtable_0.3.0 zlibbioc_1.36.0
[31] XVector_0.30.0 DelayedArray_0.16.2
[33] coop_0.6-2 kernlab_0.9-29
[35] BiocSingular_1.6.0 future.apply_1.7.0
[37] abind_1.4-5 scales_1.1.1
[39] edgeR_3.32.1 DBI_1.1.1
[41] Rcpp_1.0.6 viridisLite_0.3.0
[43] xtable_1.8-4 progress_1.2.2
[45] dqrng_0.2.1 bit_4.0.4
[47] rsvd_1.0.3 ResidualMatrix_1.0.0
[49] httr_1.4.2 ellipsis_0.3.1
[51] pkgconfig_2.0.3 XML_3.99-0.6
[53] farver_2.1.0 scuttle_1.0.4
[55] CodeDepends_0.6.5 sass_0.3.1
[57] locfit_1.5-9.4 utf8_1.2.1
[59] tidyselect_1.1.0 labeling_0.4.2
[61] rlang_0.4.10 later_1.1.0.1
[63] munsell_0.5.0 BiocVersion_3.12.0
[65] tools_4.0.4 cachem_1.0.4
[67] generics_0.1.0 RSQLite_2.2.4
[69] ExperimentHub_1.16.0 evaluate_0.14
[71] stringr_1.4.0 fastmap_1.1.0
[73] yaml_2.2.1 processx_3.4.5
[75] knitr_1.31 bit64_4.0.5
[77] purrr_0.3.4 future_1.21.0
[79] sparseMatrixStats_1.2.1 mime_0.10
[81] origami_1.0.3 xml2_1.3.2
[83] biomaRt_2.46.3 compiler_4.0.4
[85] beeswarm_0.3.1 curl_4.3
[87] interactiveDisplayBase_1.28.0 statmod_1.4.35
[89] tibble_3.1.0 bslib_0.2.4
[91] stringi_1.5.3 highr_0.8
[93] ps_1.6.0 RSpectra_0.16-0
[95] lattice_0.20-41 ProtGenerics_1.22.0
[97] Matrix_1.3-2 vctrs_0.3.6
[99] pillar_1.5.1 lifecycle_1.0.0
[101] BiocManager_1.30.10 Rdpack_2.1.1
[103] jquerylib_0.1.3 BiocNeighbors_1.8.2
[105] data.table_1.14.0 cowplot_1.1.1
[107] bitops_1.0-6 irlba_2.3.3
[109] httpuv_1.5.5 rtracklayer_1.50.0
[111] R6_2.5.0 bookdown_0.21
[113] promises_1.2.0.1 KernSmooth_2.23-18
[115] gridExtra_2.3 parallelly_1.24.0
[117] vipor_0.4.5 codetools_0.2-18
[119] assertthat_0.2.1 openssl_1.4.3
[121] sparsepca_0.1.2 withr_2.4.1
[123] GenomicAlignments_1.26.0 Rsamtools_2.6.0
[125] GenomeInfoDbData_1.2.4 hms_1.0.0
[127] grid_4.0.4 beachmat_2.6.4
[129] rmarkdown_2.7 DelayedMatrixStats_1.12.3
[131] Rtsne_0.15 shiny_1.6.0
[133] ggbeeswarm_0.6.0
Bibliography
Messmer, T., F. von Meyenn, A. Savino, F. Santos, H. Mohammed, A. T. L. Lun, J. C. Marioni, and W. Reik. 2019. “Transcriptional heterogeneity in naive and primed human pluripotent stem cells at single-cell resolution.” Cell Rep 26 (4): 815–24.